HIstogram equalization
1) 개요
- Transform color image to grayscale is the one of histogram equalization.
- This is often a good way to normalize image intensity before further processing.
- Effective way to increase image contrast.
2) 방법
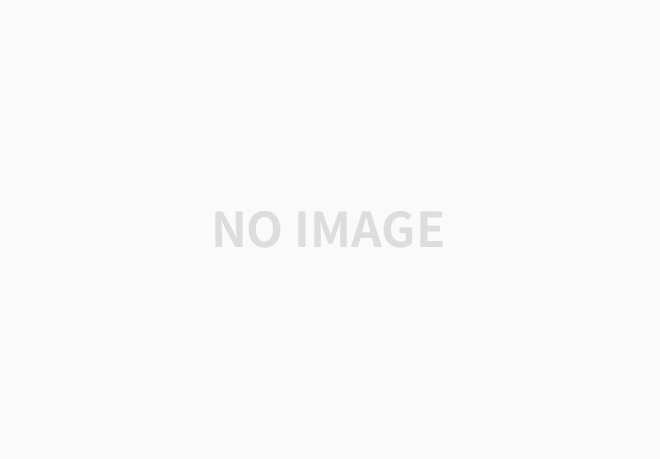
- Implement histogram of image by flattening.
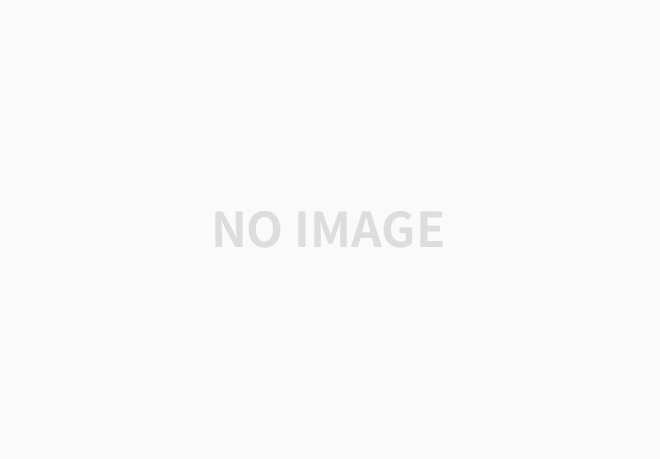
- Use cumulative distribution function (cdf) of the pixel values. (stack the frequency of pixels)
- Normalize the cdf to range between [0, 1].
- Use linear interpolation of cdf to find new pixel values.
A way of using histogram equalization equation
h(v)=cdf−cdfmincdfmax−cdfmin×255
3) Result
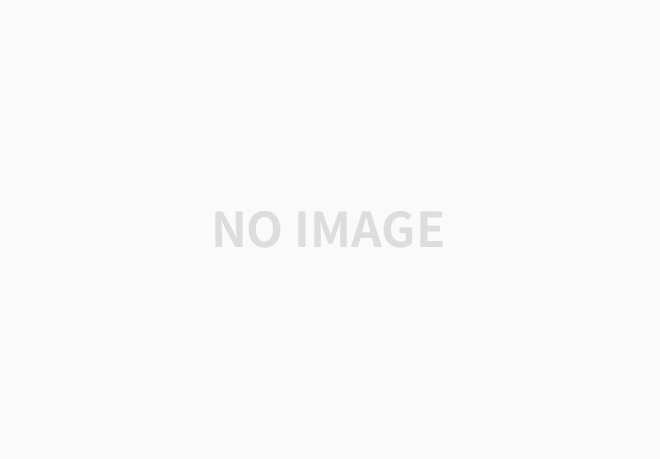
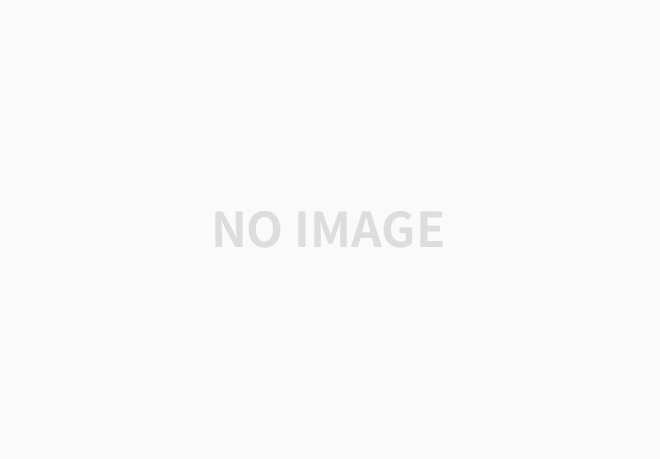
Code
# Using linear interpolation
def histeq(im,nbr_bins=256):
""" Histogram equalization of a grayscale image. """
# get image histogram
imhist,bins = histogram(im.flatten(), nbr_bins, normed=True)
# cumulative distribution function
cdf = imhist.cumsum()
cdf = 255 * cdf / cdf[-1] # normalize
# use linear interpolation of cdf to find new pixel values
im2 = interp(im.flatten(), bins[:-1], cdf)
return im2.reshape(im.shape), cdf
# Using normalization equation
img = cv2.imread('images/hist_unequ.jpg');
hist, bins = np.histogram(img.flatten(), 256,[0,256])
cdf = hist.cumsum()
# cdf의 값이 0인 경우는 mask처리를 하여 계산에서 제외
# mask처리가 되면 Numpy 계산에서 제외가 됨
# 아래는 cdf array에서 값이 0인 부분을 mask처리함
cdf_m = np.ma.masked_equal(cdf,0)
#History Equalization 공식
cdf_m = (cdf_m - cdf_m.min())*255/(cdf_m.max()-cdf_m.min())
# Mask처리를 했던 부분을 다시 0으로 변환
cdf = np.ma.filled(cdf_m,0).astype('uint8')
img2 = cdf[img]
참고